As a developer primarily focused on R, I strive to follow good development practices: writing clear user documentation with articles and examples; creating robust tests; and automating checks and deployments with CI/CD workflows. These habits have allowed me to build reliable and maintainable projects, but I asked myself: Are these practices transferable if I venture into a different ecosystem, such as Node.js?
To answer this question, I set myself a personal challenge: create a fully functional website using Node.js and JavaScript, while taking the opportunity to test GitHub Copilot Workspace as my primary development assistant. My goal was to see if Copilot could guide me from start to finish, assuming I knew nothing. I also wanted to test the hypothesis that even without deep expertise in a language or framework, success is achievable by relying on solid practices and the right tools.
This challenge was well-timed, as I had a concrete project in mind: displaying the main walking trails around my town on an interactive map to share with members of my hiking association. I also wanted the system to be easy to update by adding new trails directly from my smartphone at the end of each hike, without manually modifying the site code.
The result? An online website hosted on GitHub Pages, automatically updated with GPX files stored in my Google Drive: https://statnmap.github.io/gpx-traces-website/. This article shares the steps of the project, lessons learned, and, most importantly, how my development skills with R allowed me to successfully carry out this adventure.
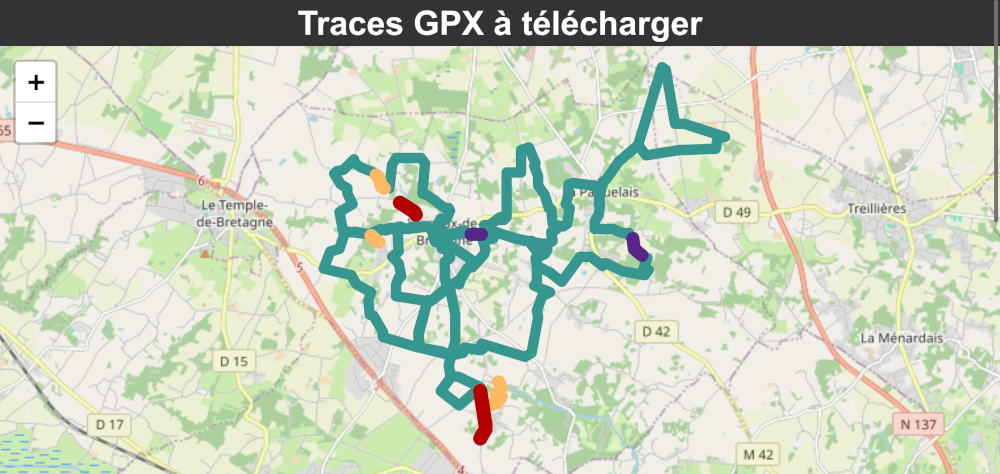
Figure 1: Snapshot of the current website
Index
Good Practices in R as a Solid Foundation
In my case, every R project is a documented, tested, and versioned package. It’s no coincidence that I developed the {fusen}
package: a tool that allows you to create an R package directly from an RMarkdown file. The goal is clear: encourage and simplify the integration of documentation and tests in R projects. This approach ensures that every project remains organized, understandable, and, most importantly, maintainable over the long term.
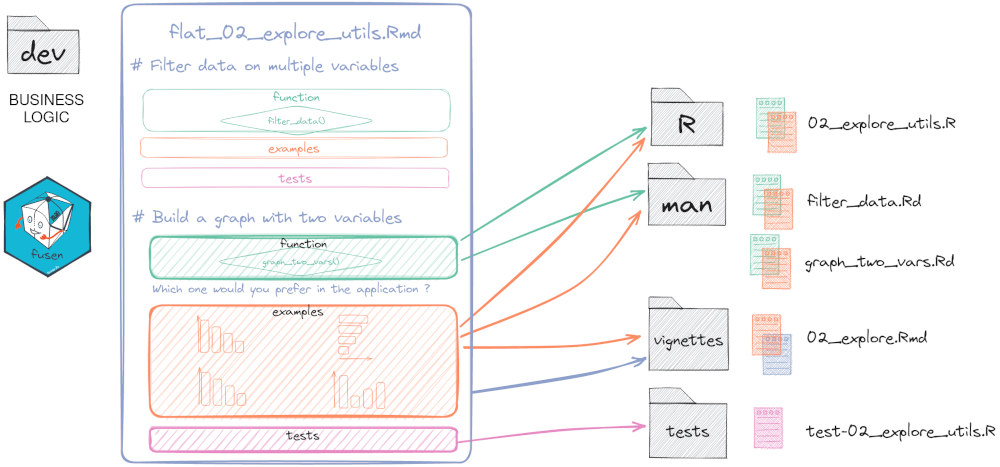
Figure 2: fusen inflate process: from Rmarkdown to full R package
These good practices, which I apply systematically, can be broken down into three main areas:
Documentation
For me, every function must come with clear and comprehensive user documentation. With{roxygen2}
, this documentation is directly integrated into the code, making it easy to update whenever modifications are made. Additionally, writing detailed articles as{rmarkdown}
vignettes provides an overview of the project or concrete usage examples.Unit Tests
Tests are not optional; they are essential to ensure that everything works as expected, both today and in the future. With{testthat}
, I ensure that each critical feature is covered. Tests also serve as implicit documentation: they demonstrate how functions should behave and help quickly identify regressions in case of changes.To illustrate their importance, I could almost create an indicator: “X days since the last time a unit test saved me.” Spoiler alert: that number remains quite low… Tests catch errors before they become problems, and believe me, they do their job!
Automation with CI/CD
Thanks to CI/CD workflows, every code modification is automatically checked: tests are run, documentation is generated, and the project is validated. This automation not only saves time but also ensures consistent quality, regardless of the number of contributors or changes made. It also allows testing the code in various environments: different operating systems, multiple R versions, or even specific configurations.For a web project like this, continuous integration goes even further by automatically deploying changes to the live website. This significantly reduces the risk of human errors and offers a smooth and secure update process. The result? Peace of mind and simplified maintenance, particularly valuable for a project that evolves regularly.
These practices, deeply rooted in my work with R, are not limited to a specific language or tool. They are universal software development principles, and this project in Node.js was the perfect opportunity to test their effectiveness in a completely different environment.
An Essential Design Phase
When I embarked on this project to create a website with Node.js and JavaScript, I began with a crucial step: user-centered design and success criteria definition. Before writing a single line of code, it was important to clearly define:
What were the main user needs?
As the primary user of the application, I had two main needs:
1. Easily view existing trails on an interactive map from my smartphone.
2. Download the desired GPX file to my smartphone to import it into my preferred hiking app (such as OutdoorActive or Komoot) and start a hike directly without prior preparation.
What criteria would define project success?
The site had to be publicly accessible, functional on smartphones, and easily maintainable. In addition to meeting the above needs, it had to allow the easy addition of new trails, ideally through an automated solution requiring no complex manipulations.
This preliminary reflection allowed me to clarify the objectives:
- Enable a clear and interactive visualization of available trails.
- Provide a simple way to download GPX files for each trail.
- Facilitate the addition of new tracks via automation, making the system adaptable without effort.
The Experience with GitHub Copilot Workspace for Technical Design
After defining the user vision, Copilot Workspace took over to turn my needs into concrete development suggestions. To push the experience to the maximum, I approached the project as if I had no expertise in web development.
A Minimum Viable Product (MVP)
My first “brainstorm” request was deliberately simple to quickly achieve a minimum viable product (MVP): (Out of habit, I talk to it in English. You can always speak to it in French; it will understand, but it will reply in English.)
A static website on github pages, updated through github actions.
The website :
- uses a list of gpx files stored in the repository as input,
- shows the gpx traces on a interactive map
- allows the users to click traces on the map to download them
- each trace has a category "sec", "humide", "boueux", allowing for selection of traces shown on the map
It is ok if the gpx traces need a preparation during website pre-build.
Development Ideas and Code Generation
Copilot Workspace then proposed several development ideas, corresponding to possible strategies to combine in order to meet my needs. At this stage, it was possible to choose:
- Generate only the plan, to clarify the project’s major steps.
- Generate complete code directly from the plan, to move faster.
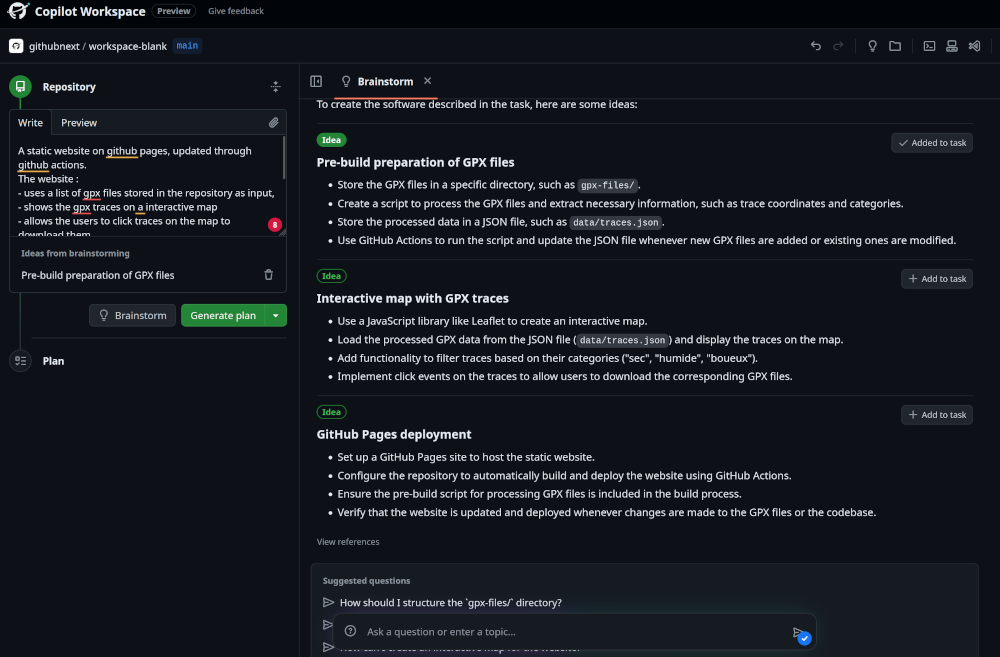
Figure 3: My instructions on the left. Brainstorm output on the right
I first chose to generate a plan before moving on to the complete code.
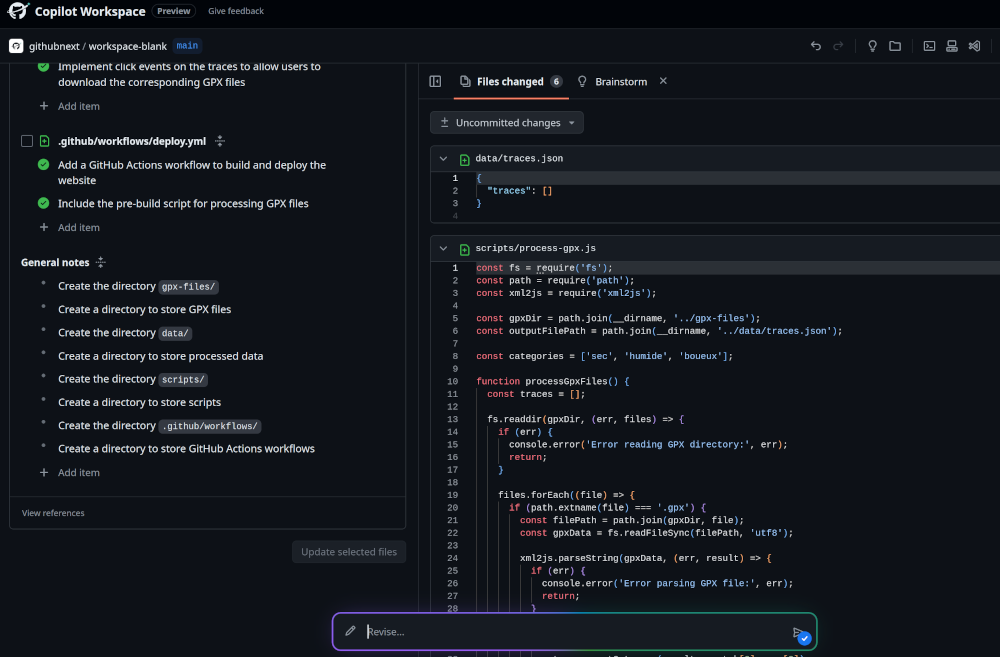
Figure 4: The plan, with actions to accomplish on the left. Code generated automatically on the right.
Next, several options were available to me:
- Request a plan review (“Brainstorm”) to add ideas or clarify certain points.
- Review the generated code (“Revise”) if optimizations were necessary.
- Modify the code myself, using my development skills.
Smooth and Guided Development
When I was satisfied with the result, I was able to create a private GitHub repository directly from Copilot Workspace, generate commits, and push the code to GitHub.
For project updates, I started new brainstorming sessions to:
- Add features or improvements.
- Fix errors detected during continuous integration.
Copilot Workspace suggested making changes in the form of Pull Requests, facilitating a structured and maintainable workflow.
Concrete Examples
Among the proposals from Copilot Workspace:
A classic structure for a web page, with folders dedicated to JS scripts, well separated according to functionalities: data preparation on one side, map interactivity on the other.
A CI/CD workflow, with steps for data preparation, site building, and deployment on GitHub Pages.
Assistance in writing the script for manipulating GPX files, to prepare them for display on the map, as well as in creating filter components on the map.
A simple solution for managing GPX file downloads, allowing users to easily retrieve tracks for their hiking application.
Valuable help in correcting the CI/CD workflow, assisting me in managing errors encountered during data preparation and site building (see screenshot).
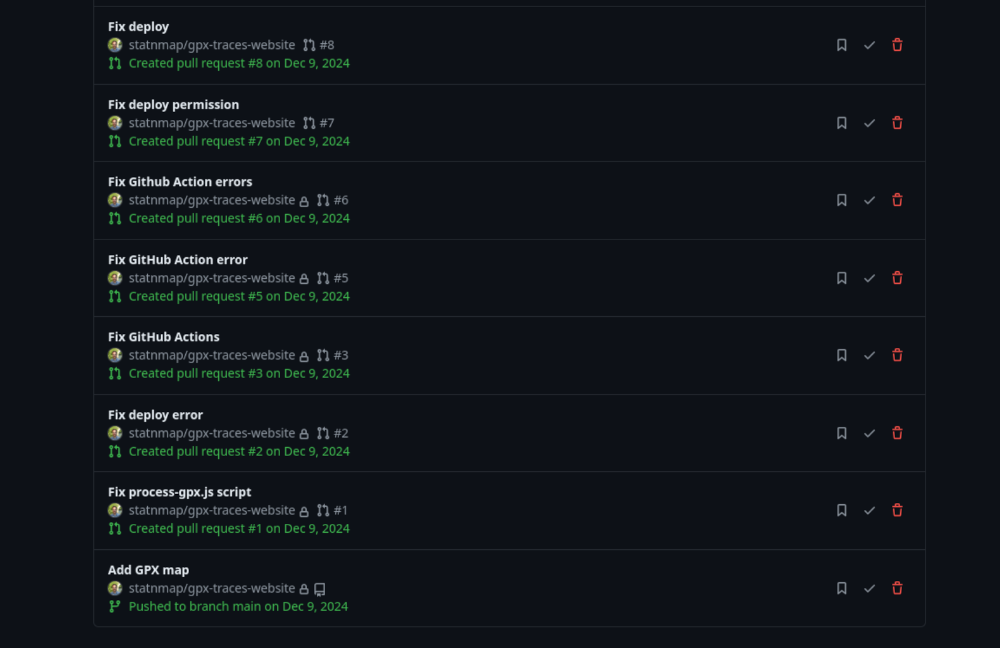
Figure 5: Multiple independant brainstorm sessions to fix different CI/CD errors faced during development.
Lessons Learned from Using Copilot Workspace
This experience reminded me of the importance of clearly defining user needs from the start in natural language. A clear and user-centered design facilitates the technical development, especially with tools like GitHub Copilot Workspace. This proves that a well-thought-out project from the beginning allows for gaining efficiency and quality throughout the process.
However, it is essential not to overlook good development practices such as documentation, testing, and automation. These practices remain solid pillars, regardless of the language or framework used, and allow for steady progress on such a project, promoting quality at every stage.
1. Copilot Workspace as a Tool for Technical Leads
Copilot Workspace is not just a simple code generator for writing or debugging a file in progress, it is a valuable tool for technical project execution.
Not only does it offer suggestions on how to approach your needs, but it also allows you to generate all the folders and files in the same interface, in one place, and at the same time. This is a significant time-saver, especially when setting up a new project. Additionally, since it is fully integrated with your GitHub repository, you can push your changes directly online.
It complements Copilot in your preferred code editor seamlessly.
2. Linking with Feature Requests
I could have gone even further by writing my needs as GitHub issues, with well-structured user stories and success criteria. I tested this on two issues to see. These issues can be opened directly in the Workspace, automatically generating “brainstorms”. Linking these issues to commit messages also improves project traceability. For tracking project progress with other developers, this is a valuable asset.
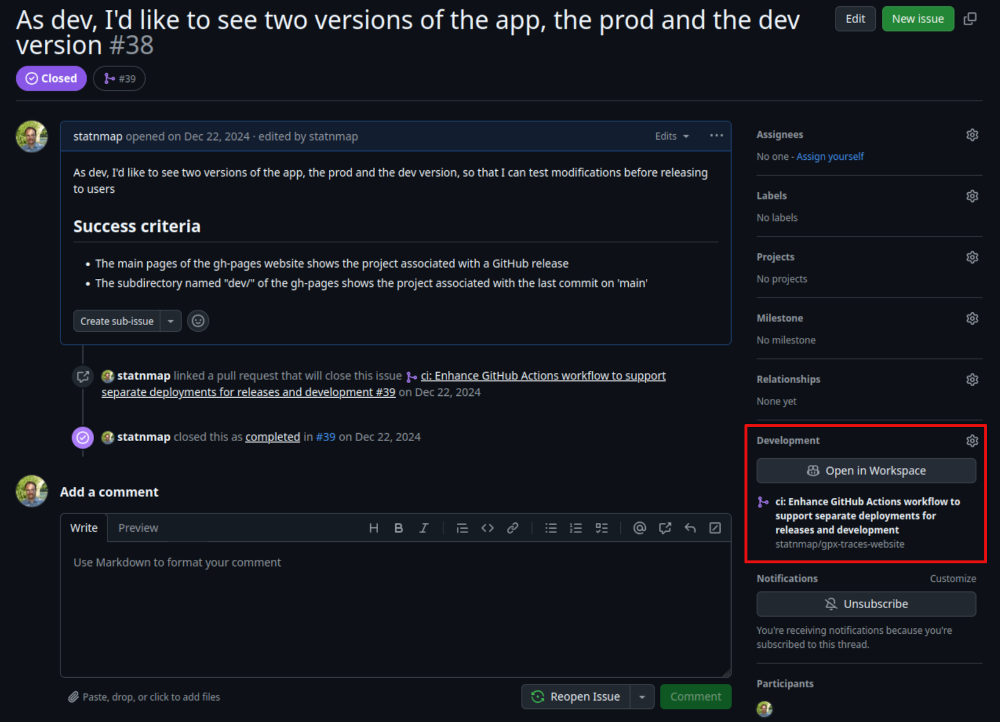
Figure 6: Screenshot of a GitHub issue with the button to open it directly on GitHub Workspace
2. The Importance of CI/CD from the Start
From the beginning, I deliberately forced Copilot to set up a CI/CD workflow. Even though I didn’t need to dive deeply into the JavaScript code, I was able to have functional code simply by asking it to fix the errors encountered in the PRs: “Here is the CI error message, can you help me fix it?”.
The time saved was considerable, especially for a language I am less familiar with. This choice confirmed a truth I already know from my R projects: CI/CD-related errors are often the most time-consuming and complex to debug, as we don’t always think about the specifics of a clean or different OS from our workstation…
I am therefore not surprised that an AI struggles to propose a functional CI/CD workflow on the first try. However, it was of great help in fixing these errors and making the pipeline reliable.
3. Documentation and Tests: Leaving Nothing Out
By default, Copilot did not suggest unit tests or function documentation. Thanks to my development habits with R, I made sure to quickly integrate unit and end-to-end tests to ensure that the outputs of the JS functions were consistent with user needs.
Similarly, my need for documentation (inherited from {roxygen2}) led me to ask it to document the functions and generate a documentation site, like {pkgdown}, with JSDoc: https://statnmap.github.io/gpx-traces-website/docs/. This approach made the project more robust and maintainable.
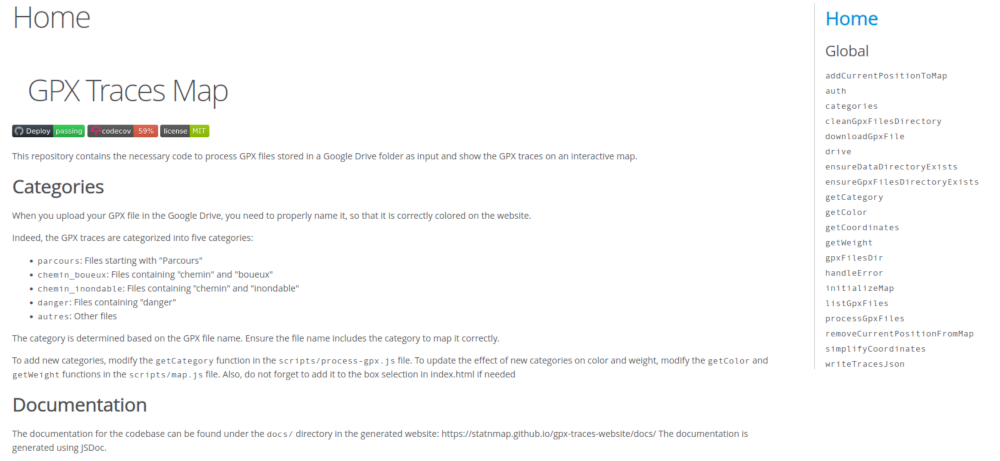
Figure 7: Documentation webpage of the project from JSDoc
4. Copilot learns from your practices
Once unit tests, function documentation, and README completion were set up, Copilot continued to suggest them for each project evolution. This also works with GitHub Copilot when you use it in VSCode.
Moreover, for Copilot (in general), working in a single Notebook file like with {fusen}, where documentation, code, and tests are centralized, greatly simplifies the AI’s job for code generation assistance… Just saying!
Note that this also works with commit messages. In Workspace, when you are ready to commit, Copilot can suggest automatic message generation. Similarly, VSCode locally offers this feature. If you use “conventional commit”, you will need to modify the first message, but theoretically, the following ones will retain your habits.
5. Managing Node Libraries
When adding Node.js libraries to your project, Copilot does not fully manage them automatically. You may need to manually add them to the package.json
file and especially install them with npm install
.
The good news is that Copilot Workspace often suggests the appropriate command in the last “Commands” section of the project steps. You can execute it directly in the integrated terminal by clicking on the execution icon next to the command. This saves you from having to bring the project to your local machine just to install the libraries.
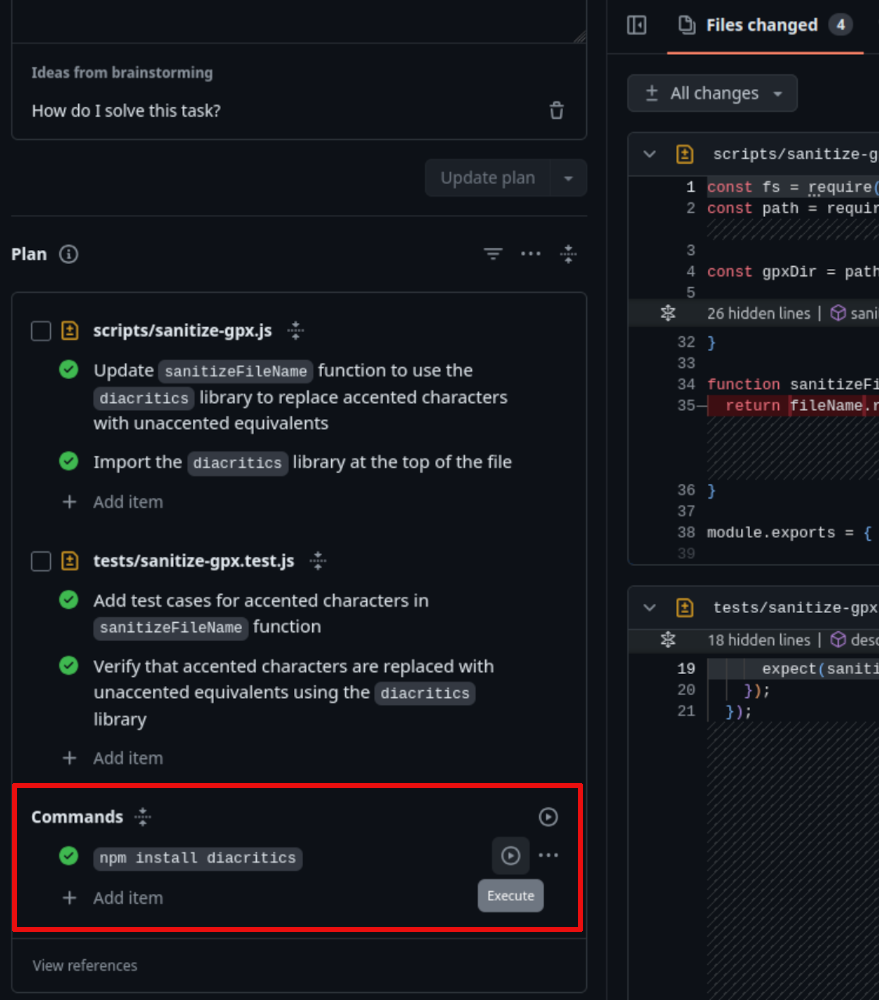
Figure 8: Section ‘Commands’ lists all commands to be manually executed, and this can be run directly in the Copilot Workspace Terminal
Version Management: Be Vigilant
One point to watch out for: dependency version management. Copilot does not always suggest the latest version in package.json
. Additionally, it does not suggest updating dependencies or resolving version conflicts. You will need to be vigilant on this point.
Fortunately, continuous integration will quickly alert you if a dependency causes problems! And to anticipate issues before committing, consider using the Copilot Workspace Terminal to run commands like npm audit
.
Get Started!
Programming languages may seem different, but they are quite similar. Developers draw inspiration from each other and evolve their languages based on what works well elsewhere. Of course, when switching languages, you will need to learn new syntax, libraries, and ways of doing things. But good development practices are universal.
This project in Node.js allowed me to verify this. By relying on my habits acquired with R, I was able to design, develop, and deploy a complete website without (almost) writing a single line of code. Copilot Workspace guided me at every step, but it was indeed my development skills and habits that made the difference.
I encourage you to explore new technologies, step out of your comfort zone, while keeping in mind that the solid foundations you have acquired with R or any other language are transferable. Tools evolve, languages change, but good practices remain. And that is the strength of any developer.
You can find :
- the source code of the project on GitHub: gpx-traces-website.
- the website online: https://statnmap.github.io/gpx-traces-website/.
- documentation generated directly with CI/CD using JSDoc: https://statnmap.github.io/gpx-traces-website/docs/.
And you? What has your experience been like with GitHub Copilot Workspace or another development assistant? What are your tips for successfully tackling a project in a new language or framework? Share your feedback and tips in the comments!
Citation:
For attribution, please cite this work as:
Rochette Sébastien. (2025, Jan. 27). "Creating a Node.js Website with GitHub Copilot Workspace Using Best Development Practices in R". Retrieved from https://statnmap.com/2025-01-27-creating-a-website-in-node-js-with-github-copilot-workspace/.
BibTex citation:
@misc{Roche2025Creat,
author = {Rochette Sébastien},
title = {Creating a Node.js Website with GitHub Copilot Workspace Using Best Development Practices in R},
url = {https://statnmap.com/2025-01-27-creating-a-website-in-node-js-with-github-copilot-workspace/},
year = {2025}
}